Add a non-ROS data stream
You can ingest non-ROS data using the Formant Agent SDK. The Agent SDK is a lightweight Python module that allows you to run scripts on your device to gather custom data and ingest it into Formant. You can then use the full suite of Formant functionality with that data.
Documentation and SDK reference for the Agent SDK, alongside other other APIs and SDKs, can be found in the API/SDK reference section of our site.
This guide will teach you how to configure a data stream using the Agent SDK.
Step 1: Install the Formant Agent SDK
You can find instructions to install the Formant Agent SDK here: Agent SDK installation and overview.
Step 2: Write an Agent SDK script which ingests data
You can find instructions and a simple example for data ingestion here: Ingesting basic datapoints.
For a full reference of the Agent SDK, see Agent SDK Reference.
When you run your application which ingests data to a stream, that stream will be created in the Formant UI automatically. If you don't see this stream, or if you want to configure the stream manually in the Formant UI, proceed to Step 3.
Step 3: Add an API stream
Once your device is ingesting data from the Agent SDK, you'll want to add an API stream to visualize and utilize that data in Formant.
- In Formant, in the upper-left corner, open the menu and click Settings.
- Click Devices, and then click on the device you want to configure.
- Click the Telemetry tab, and then click Add Stream.
- Click API. This will open the New API Stream configuration window:.
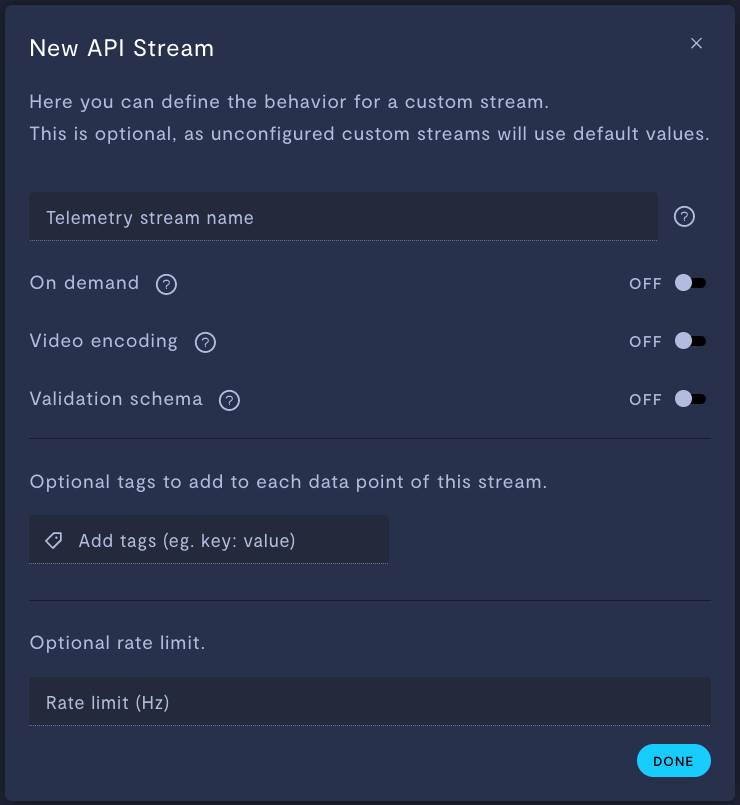
Property | Usage |
---|---|
Telemetry stream name | Give the stream a name. |
On demand | If ON, this stream will store data locally on your device, and only upload data to Formant when you click Retrieve on-demand data. Rate limit controls the rate at which datapoints are added to the on-device buffer. For more information, see On demand streams.If OFF, this stream will ingest periodically. Rate limit controls the rate of data ingestion. |
Video encoding | Only applicable for sensor_msgs/Image and sensor_msgs/CompressedImage streams. Choose whether you want images ingested on this stream to be encoded as a video. |
Validation schema | Only applicable for JSON and text streams. Choose a configuration schema for this stream. |
Add tags (e.g. key: value ) (optional) | Add tags to each datapoint of this stream. For more information on tags, see Configure access levels. |
Rate limit (Hz) (optional) | If On demand is ON: Set the rate at which data is added to the on-device buffer. Data in the on-device buffer will be ingested by the agent when you click Retrieve on-demand data. For more information, see On demand streams.If On demand is OFF: Set the rate at which data is ingested.The default rate is 0.5 Hz. |
Step 4: Add stream to a view
Once you've added a stream to your device, your next step is to add it to a view in Formant.
Add the streams you want to visualize, and then move on to Viewing data in Formant.
If you notice an issue with this page or need help, please reach out to us! Use the 'Did this page help you?' buttons below, or get in contact with our Customer Success team via the Intercom messenger in the bottom-right corner of this page, or at [email protected].
Updated about 1 year ago