You can use the Cloud SDK to query telemetry stream data for analysis. Telemetry stream data must be ingested by the Formant agent before it can be queried. For more information on data ingestion, see Data ingestion from a device and Data ingestion from anywhere.
Querying data from the command line
If you want to query data directly from the command line, you'll want to use the
fctl
tool. For more information onfctl
, see Installing and credentialing fctl.
Query syntax and parameters
For a code sample which shows query syntax and relevant parameters, see the following example on our GitHub page: GitHub: query.py
.
Required parameters
You'll notice that the query contains start
and end
parameters. If a query contains only those two parameters, all telemetry datapoints from all devices in your organization whose timestamps fall between start
and end
will be returned. As seen in the above example, you'll want to use other parameters, such as device ID or tags, to narrow down your query response.
Timestamp formatting
start
and end
are RFC3339 formatted timestamps. (e.g. 2021-01-01T01:00:00.000Z
)
All timestamps are UTC. When using the Cloud SDK, it may be useful to toggle UTC timestamps in the Formant web application.
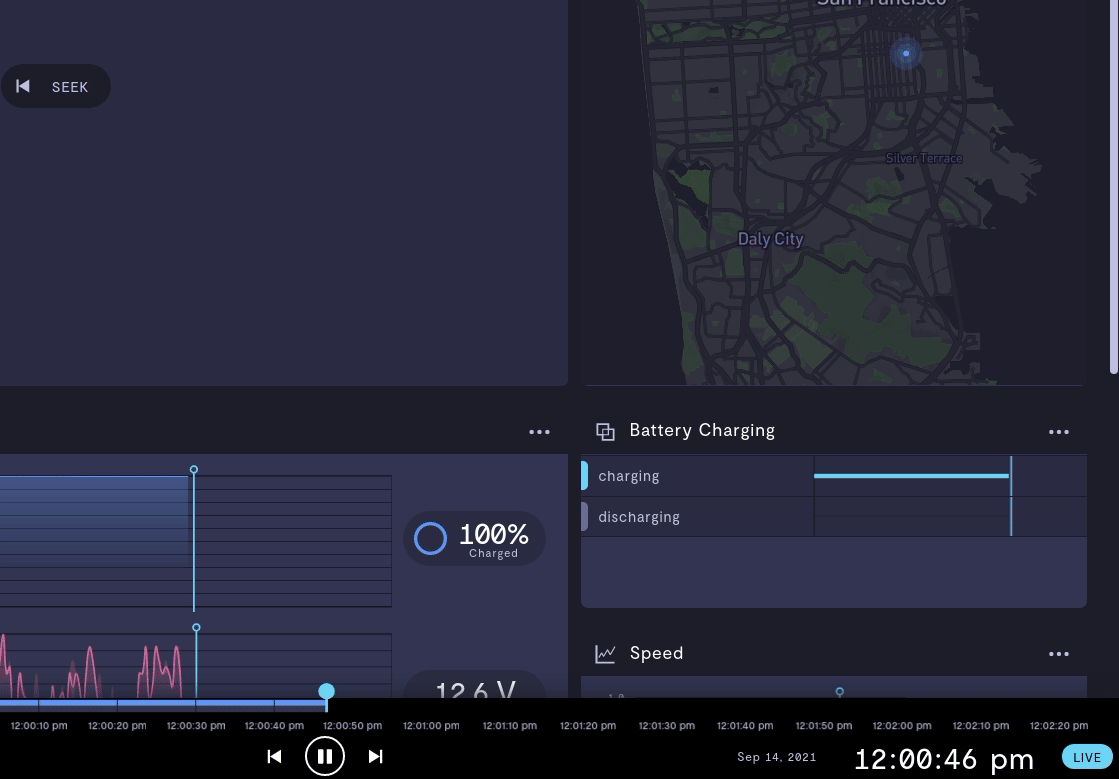
Toggling UTC timestamps may make life easier while using the Cloud SDK.
Other parameters
To further narrow down your query, you can use the optional query parameters:
Query parameter | Usage |
---|---|
deviceIds | Filter by device ID. |
names | Filter by device name. |
notNames | Exclude devices with this name. |
types | Filter by stream type. For a complete list of stream types, see How telemetry streams work. |
tags | Filter by tags applied to the device. |
Queries can be too large
Your query may be unsuccessful if you request too much data. If this happens, you'll see the following error:
requests.exceptions.HTTPError: 413 Client Error: Payload Too Large for url: https://api.formant.io/v1/queries/queries
If this happens, try narrowing your query further by applying more parameters, or by narrowing your time range.
If you notice an issue with this page or need help, please reach out to us! Use the 'Did this page help you?' buttons below, or get in contact with our Customer Success team via the Intercom messenger in the bottom-right corner of this page, or at [email protected].