Creating events
Use the create_event
method of the Client
object to create and ingest an event.
from formant.sdk.agent.v1 import Client as FormantClient
if __name__ == "__main__":
fclient = FormantClient()
fclient.create_event(
"Synchronized transporter annular confinement beam to warp frequency 0.4e17 hz",
notify=True,
tags={"Region": "North"},
severity="warning", # one of "info", "warning", "error", "critical"
)
print("Successfully created event.")
If you run the code above, you'll see an event appear on the timeline and in the events list for that device.
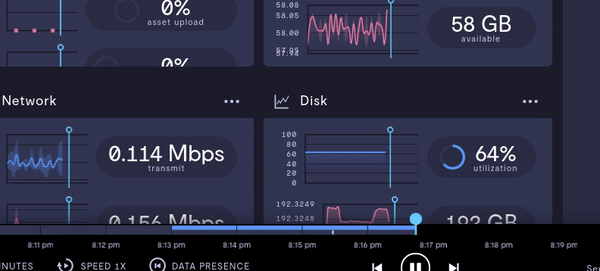
The event appears on the Formant timeline
The first argument is the text payload of the event, referred to as the event's "message".
The notify
keyword argument triggers a Formant notification if it's set to True
. The default is False
.
The severity
keyword argument must be one of "info"
, "warning"
, "error"
, or "critical"
, and determines how the event appears in Formant. The default is "info"
.
For example, fclient.create_event("Navigation in progress...")
will post an INFO severity event which does not notify users of Formant, but still appears on the timeline.
Creating an event that spans a time range
Sometimes you want to denote a region of time wherein some event was occurring. For instance, a mission, job, or tricky navigation. By using a time range event, engineers can root-cause faster and data scientists can quickly learn when all activity of a certain type was happening with a device.
To create a time range event, specify the start time via the timestamp
keyword argument, and the end time via the end_timestamp
argument.
Here's an example script that creates an event spanning 5 seconds.
import time
from formant.sdk.agent.v1 import Client
if __name__ == "__main__":
fclient = Client()
start_time = 1000 * int(time.time())
time.sleep(5.0)
fclient.create_event(
"Slept for 5 seconds",
timestamp=start_time,
end_timestamp=1000 * int(time.time()),
)
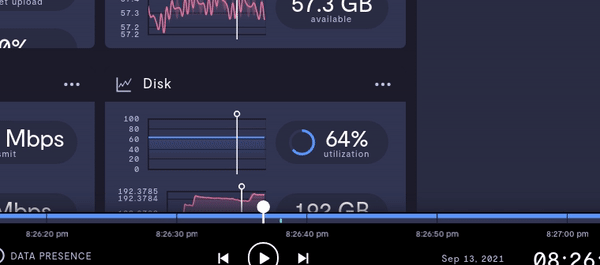
Time range events show the range they span on the device page timeline
If you notice an issue with this page or need help, please reach out to us! Use the 'Did this page help you?' buttons below, or get in contact with our Customer Success team via the Intercom messenger in the bottom-right corner of this page, or at [email protected].